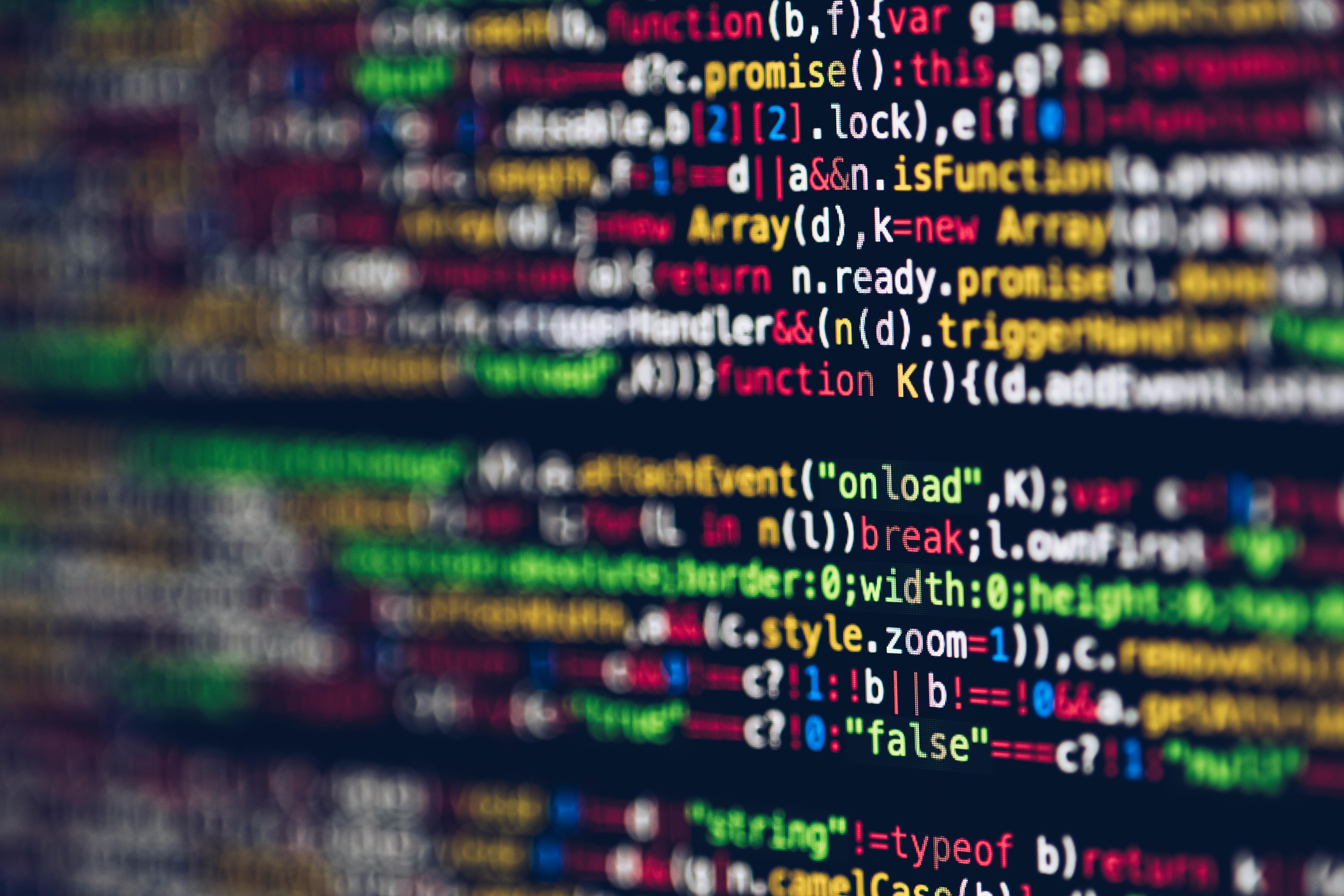
The Reality of AI in QA Testing: Beyond the Hype
Discover how AI is actually being used in QA today, focusing on real applications and practical benefits, not just futuristic promises.
Expert perspectives on AI-powered testing and quality assurance from our team of embedded QA engineers
Discover how AI is actually being used in QA today, focusing on real applications and practical benefits, not just futuristic promises.
Discover how AI is actually being used in QA today, focusing on real applications and practical benefits, not just futuristic promises.
Learn the best practices for using Cursor in Ask mode to generate effective test cases quickly.
Step-by-step guide to creating intelligent test data generation systems using AI and your schema.
How AI is augmenting manual testers' capabilities without replacing human insight.
Transform your bug documentation process with AI-generated reports that save time and improve clarity.
A practical guide to automating AI-powered testing in your continuous integration workflow.